useRef
useRef는 특정DOM을 선택해야하는 상황에서 사용을 합니다.
특정 엘리먼트의 크기를 가져온다거다나 스크롤바의 위치를 가져오거나 설정을 해야할때 useRef를 사용합니다.
자세한 설명은 React 공식 문서에서 확인 가능합니다.
https://ko.reactjs.org/docs/hooks-reference.html#useref
useRef 사용해보기
User.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React, { useState, useRef } from 'react'; | |
const User = () => { | |
const [inputs, setInputs] = useState({ | |
name: '', | |
nickname: '' | |
}); | |
const nameInput = useRef(); | |
const { name, nickname } = inputs; // 비구조화 할당을 통해 값 추출 | |
const onChange = e => { | |
const { value, name } = e.target; // 우선 e.target 에서 name 과 value 를 추출 | |
setInputs({ | |
...inputs, // 기존의 input 객체를 복사한 뒤 | |
[name]: value // name 키를 가진 값을 value 로 설정 | |
}); | |
}; | |
const onReset = () => { | |
setInputs({ | |
name: '', | |
nickname: '' | |
}); | |
nameInput.current.focus(); | |
}; | |
return ( | |
<div> | |
<input | |
name="name" | |
placeholder="이름" | |
onChange={onChange} | |
value={name} | |
ref={nameInput} | |
/> | |
<input | |
name="nickname" | |
placeholder="닉네임" | |
onChange={onChange} | |
value={nickname} | |
/> | |
<button onClick={onReset}>초기화</button> | |
<div> | |
<b>값: </b> | |
{name} ({nickname}) | |
</div> | |
</div> | |
); | |
} | |
export default User; |
App.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
import React from "react"; | |
import User from "./components/User"; | |
const App = () => { | |
return( | |
<User/> | |
) | |
} | |
export default App; |
초기화 버튼을 눌렀을때 이름 input에 포커스가 잡히도록 하는 예제입니다.
이번 예제는 velopert님의 예제를 참고했습니다.
https://velopert.com/
VELOPERT.LOG
react-redux v7.1 alpha 에 드디어 Hooks 기능이 지원되었습니다. 아직 alpha 이기에 프로덕션에서 사용하기엔 아직 이르지만 한번 사용법을 알아봅시다. 정식 릴리즈 때 많은 변화가 없었으면 좋겠네
velopert.com
실행화면.
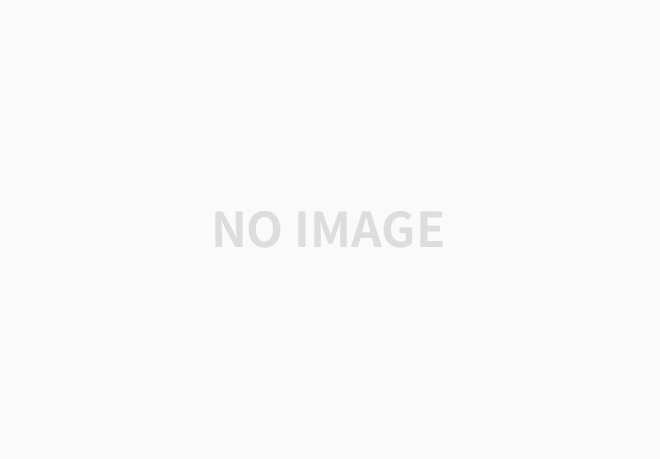
'웹 프로그래밍 > React' 카테고리의 다른 글
[React] useReducer (0) | 2021.10.18 |
---|---|
[React] useEffect (0) | 2021.10.15 |
[React] useCallback (0) | 2021.10.13 |
[React] useState (0) | 2021.10.12 |
[React] 외부 라이브러리 없이 캘린더 구현 (0) | 2021.10.11 |